Departmental Standards
Company-Wide
eCornell Styleguide & Branding
Cornell University Branding
Writing and Editing Style Guide
Faculty and Expert Naming Conventions in Courses
Cornell School and Unit Names
Tips for Campus Engagements
LSG
Legal Policies
CSG
Updating Wrike Due Dates
Photography Style Guide
eCornell Mini Visual Style Guide
The Pocket Guide to Multimedia Design Thinking (*as It Pertains to Your Job Here)
Creative Services (CSG) Handbook
Administrative
LSG Meeting Recordings and Notes
Sending Faculty Sign-Off Forms in Adobe Sign
Weekly Faculty Status Emails
Animation/Motion Design
Instructional Design
Required Course Elements
The Pocket Guide to Instructional Design Thinking at eCornell
Adding AER to Canvas
Grading
D&D Newsletter
LSG Newsletter (LSGN) - February 2024
LSG Newsletter (LSGN) - March 2022 Edition
LSG Newsletter (LSGN) - December 2023
LSG Newsletter (LSGN) - October 2021 Edition
LSG Newsletter (LSGN) - June 2022 Edition
D&D Newsletter November 2024
LSG Newsletter (LSGN) - August 2022 Edition
LSG Newsletter (LSGN) - June 2023
LSGN Newsletter April 2023
LSG Newsletter (LSGN) - February 2022 Edition
LSG Newsletter (LSGN) - October 2022 Edition
LSGN Newsletter February 2023
LSGN Newsletter March 2023
D&D Newsletter September 2024
LSG Newsletter (LSGN) - August 2023
LSG Newsletter (LSGN) - March 2024
LSG Newsletter (LSGN) - April 2022 Edition
D&D Newsletter - August 2024
LSGN Newsletter January 2023
LSG Newsletter (LSGN) - October 2023 article
LSGN Newsletter (LSGN) - April 2024
LSG Newsletter (LSGN) - November 2021 Edition
D&D Newsletter February 2025
LSG Newsletter (LSGN) - January 2022 Edition
LSGN Newsletter December 2022
D&D Newsletter April 2025
LSG Newsletter (LSGN) - July 2022 Edition
LSG Newsletter (LSGN) - September 2022 Edition
Course Development
Image Uploads for Inline Projects
How to Install the Firefox Canvas Utilities Extension
Revising a Course/ Creating a Redux Version/ Course Updates
Creating a Perma Link With Perma.cc
Course Content Deletion Utility — Removing All Course Content
Teleprompter Slide Template
Course Names
Requesting High Resolution Video Uploads
Technical Talking Points Template
Online Resources in Credit-Bearing Courses
Hiring Actors for an eCornell Project
Marketing
Operations
Tech
Master Course Template Differences (8675309s)
Doc-Based Master Course Template and Standards (8675309-DOC)
Pedagogical Guidelines for Implementing AI-Based Interactives: AER
Coding Master Course Template and Standards (8675309-CODE)
Hero Image
Platform Training
Administrative Systems
ADP
Google Drive
Downloadables Process
Embed a Document from Google Drive
Adding Google Links to Canvas
File Naming and Storage Convention Standards
Google Drive for Desktop Instructions
Storing Documents in Multiple Locations
Wrike
Wrike System Fundamentals
Field Population
1.0 to 2.0 Wrike Project Conversion
Blocking Time Off in Work Schedule (Wrike)
Wrike Custom Field Glossary
Wrike "Custom Item Type" Definitions
How to Create a Private Dashboard in Wrike
Using Timesheets in Wrike
Importing Tasks into a Wrike Project
Wrike Project Delay Causes Definitions
Setting OOO Coverage for Roles in Wrike
How to Change a Project's Item Type in Wrike
Using Search in Wrike
How to Create a Custom Report in Wrike
@ Mentioning Roles in Wrike
Automate Rules
Using Filters in Wrike
Managing Exec Ed Programs in Wrike
External Collaborators
Wrike for External Collaborators: Getting Started
Wrike for External Collaborators: Views
Wrike for External Collaborators: Tasks in Detail
Wrike Updates
New Experience Update in Wrike
Wrike Course Development Template 2.0 - What's New
Wrike - Course Development Template 3.0 Release Notes
Wrike Process Training
Course Development & Delivery Platforms
Canvas
Development
Adding Custom Links to Course Navigation
Adding Comments to PDFs from Canvas Page Links
Setting Module Prerequisites and Requirements in Canvas
Canvas Page Functionality
Create a New Course Shell From 8675309
Using LaTeX in Canvas
Search in Canvas Using API Utilities - Tutorial
Reverting a Page to a Previous Version
Student Groups
Create Different Canvas Pages
Importing Specific Parts of a Canvas Course
Canvas HTML Allowlist/Whitelist
Understanding Canvas Customizations/Stylesheets
Operations
Discussion Page Standards
How to import a CU course containing NEW quizzes
Canvas LMS: NEW Quiz compatibility
Faculty Journal
Course Content Style Guide
Click-To-Reveal Accordions in Canvas
Course Maintenance Issue Resolution Process
Meet the Experts
Codio
Codio Operations
Managing Manually Graded “Reflect and Submit” Codio Exercises
Codio Structure and Grading for Facilitators
Premade Codio Docs for Ops & Facilitators
Codio Remote Feedback Tools for Facilitators
Developers
Development Processes
Creating a New Codio Course
Creating a New Codio Unit
Integrating a Codio Course into Canvas
Embedding a Codio Unit into Canvas
Setting Up the Class Fork
Setting Up the Class Fork (LTI 1.3)
R Studio - Exclusion List for R Code
Mocha/Selenium Autograding
Starter Packs in Codio
Configuring Partial Point Autograders in Codio
Launch a Jupyter Notebook from VM
Program-Specific Developer Notes
Codio Functionality
Jupyter Notebooks
Jupyter Notebooks - nbgrader tweaks
Jupyter Notebooks Style Guide
Adding Extensions to Jupyter Notebooks
Setting up R with Jupyter Notebooks
Change Jupyter Notebook Auto Save Interval
How to Change CSS in Jupyter Notebook
RStudio in Codio
How To Centralize the .codio-menu File to One Location
Codio Fundamentals for LSG
Using the JupyterLab Starter Pack
Using Code Formatters
Using the RStudio Starter Pack
Conda Environments in Codio
Updating Codio Change Log
eC Facilitator Guide to Codio
Migrating to Updated Codio Courses
Qualtrics
Ally
Ally Institutional Report Training
Ally Features Overview Training
Using the Ally Report in a Course
Ally Vendor Documentation/Training Links
Adobe
Other Integrations
H5P
Modifying Subtitles in H5P Interactive Videos
Embedding H5P Content Into Canvas
Troubleshooting H5P Elements in Canvas
Inserting Kaltura Videos into H5P Interactive Videos
Adding Subtitles to H5P Interactive Videos
S3
BugHerd
Instructional Technologies & Tools Inventory
Canvas API Utilities
Getting started with the MOP Bot
eCornell Platform Architecture
HR & Training Systems
Product Development Processes
Accessibility
What Is Accessibility?
What Is Accessibility?
Accessibility Resources
Accessibility Considerations
Accessibility Support and Assistive Technology
Structural Accessibility
Accessibility Design and Development Best Practices
Accessible Images Using Alt Text and Long Descriptions
Accessible Excel Files
Accessibility and Semantic Headings
Accessible Hyperlinks
Accessible Tables
Creating Accessible Microsoft Files
Mathpix: Accessible STEM
Design and Development General Approach to Accessibility
Integrating Content Authored by a Third Party
Planning for Accessible Tools
Accessibility Considerations for Third Party Tools
Studio Accessibility
Designing for Accessible Canvas Courses
Accessibility: Ongoing Innovations
Course Development
Planning
Development
0. Design
1. Codio Units
1. Non-Video Assets
3. Glossary
4. Canvas Text
4. Tools
4. Tools - Wrike Task Definitions
3. Review And Revise Styled Assets
ID/A to Creative Team Handoff Steps
General Overview of Downloadables Process
Course Project: Draft and Final
Excel Tools: Draft and Final
eCornell LSG HTML Basics
1. Non-Video Assets - Wrike Task Definitions
2. Video
Multifeed Video
2. Video (Standard) - Wrike Task Definitions
Studio Tips
Tips for Remote Video Recording Sessions
Who to Tag for Video Tasks
3. Animation
3. Animation - Wrike Task Definitions
2. Artboard Collab Doc Prep
6b. Motion Design Review and Revise
Who to Tag for Animations Tasks
3. Artboard Collab Process Walkthrough
DRAFT - FrameIO Process Walkthrough
Motion Contractor Guide for IDAs / IDDs
Requesting / Using Stock Imagery (Getty Images and Shutterstock)
3. Ask the Experts
5. On-Demand Conversion
1. Write Content for On-Demand
On-Demand: Conversion Notes
On-Demand: Writing Quiz Questions
On-Demand: Writing Blended Learning Guides (DRAFT)
On-Demand: Lesson Description and Objectives (DRAFT)
2. Build On-Demand Lesson
On-Demand: Create a Blended Learning Guide (BLG)
On-Demand: Create Lesson Shells in Canvas
On-Demand: Populate Homepage Content
On-Demand: Add Quiz Assessment Content
On-Demand: Reformat Wrap-Up
On-Demand: Prepare Lesson for QA
On-Demand: Request Banner Image
OD Updates Process
5. On-Demand Conversion - Wrike Task Definitions
5. Review
5. Review - Wrike Task Definitions
1. Prep Course for Reviews
2. Conduct Student Experience Review
3. Implement Creative Director Edits
3. Implement IDD Edits
3. Implement Student Experience Review Edits
4. CSG - Revise Tools Export 1
5. Conduct Faculty Review
6. Implement Faculty Edits
7. Conduct Technical Review of Course (STEM-only)
2. Conduct IDD or Sr ID Review
6. Alpha
6. Alpha - Wrike Task Definitions
Alpha Review Process
Prepare a course for Alpha review
Schedule & Conduct Alpha Triage Meeting
7. QA
7. QA - Wrike Task Definitions
1. Complete Dev to QA Checklist
2. Copy Edit Captions
2. Copy Edit Course & Files
4. Conduct Content QA of Course
4. Final Creative Review and Export
Adding Chat With Tech Support to Course Navigation
5. Implement QA Edits
Working With Video Captions That Contain Special Characters
Copy Edit Captions in SubPLY
Creating a Course Style and Settings Guide
Copy Editing Content in Frame.io
Copy Edit Captions in 3Play
Tag a Video for Transcription by 3Play
Course QA Checklists
8. Deployment
8. Deployment - Wrike Task Definitions
1. Finalize Master Version of Course
2. Create & Add Course Transcript (CT) to Course
Replace a Master -M With a Redux Version of the Course
3. Create -T (Training Course) and Associate With Master Blueprint
Canvas Blueprint Course Functionality
Project Management in Wrike
Managing Project Reporting in Wrike
Managing Task Needs/Schedule in Wrike
Adding Tasks
Comments and Communication
Statuses
Updating Task Start and Due Dates
Predecessors
Durations
Rollups
Calculating Project Schedule by Deadline in Wrike
Creating Course Project Plans in Wrike
Setting Custom Capacity for Resources
Customizing Effort in a New Project Plan
Marking Projects Complete in Wrike
How to Set Up Workload Charts to Track Effort in Wrike
For-Credit Considerations
1-Sheet Population
Post-Development
Program Facilitation & Operational Guidelines
Data Science
Facilitator Resources
Canvas Navigation
Adding Events to the Course Calendar
Navigating Canvas and the Dashboard
How Do I View Previous Courses I Have Taken or Facilitated?
Why Am I Receiving Duplicate Canvas Emails?
How Do I Edit My Canvas Profile?
Send Students a Direct Message on Canvas
Adding Notes to Canvas Gradebook
How Do I Send Students Nudges from the Gradebook?
Overview of 2024 Changes- Course Layout and Structure
How Can I Update My Canvas Notification Preferences?
Ursus Navigation
How Do I Access My Offer in Ursus?
How Do I Edit My Ursus Profile?
How Do I Request Time Off (Blackout Dates)?
How Can I Request to Learn More Courses?
Live Sessions
When Should I Schedule My Live Sessions For?
Changing Live Session Date and Time After Created with Facil Tool
Help! I Need to Reschedule a Live Session
Live Session Information page
Set up Live Sessions with the Facil Tool
Combining Live Sessions with Facil Tool
Course Set-Up
Course Set Up: Getting Ready for Live Sessions
Recording and Posting a Welcome Video
Course Set Up: When can I begin to edit my course?
Course Set Up: Reviewing Due Dates
Course Set Up: Reviewing Announcements
What Do I Need to Do to Make Sure My Course is Set Up Correctly?
How Do I Customize My Course Sections?
Course Announcements and Messages Templates
Facilitator Focus
Zoom and other Technical Support
How Do I Set Up My Zoom Account?
Support Resources for Facilitators
Zoom Features: Preparing for Live Sessions
How to Upload Videos to Zoom On-Demand
Student Survey FAQs
How Do I Save and Refer Back to Zoom Recordings?
How Do I Find My Personal ID Meeting link in Zoom?
Benefits as an eCornell Employee
Do I Have Access to Microsoft Office as a Cornell Employee?
Taking Courses as a Student
Professional Development Benefit
Student Success
Help! My Students Can't View a Video Within My Course
Extensions and Retakes
Policies and Navigation Resources for Students
Students enrolled through special groups: Corporate and VA
Unique Circumstances for Student Extensions and Retakes
Can I Provide Students with a Letter of Recommendation?
Understanding and Addressing Instances of Plagiarism
Support Resources for Students
Understanding and Addressing Use of AI
Help! My Student is Having a Hard Time Uploading a Video
Unique Student Situations
I Have a Student Requesting Accommodations- How Should I Proceed?
Canvas Mobile App
Messaging Students via the Canvas Mobile App
Setting up and Navigating the Canvas Mobile app
Setting up Push Notifications on Canvas Mobile
Canvas App Features for eCornell courses
New Facilitator Onboarding and Quick References
Facilitator Onboarding at eCornell
Why Do I Have Multiple eCornell email and Canvas Accounts?
Quick reference: Systems and Accounts we use at eCornell
Quick reference guide: Key eCornell Personnel
How do I log Onto Canvas and Access FACT101?
How Do I Add eCornell to My Email Signature?
Getting the Most Out of Learning Assignments
What to Expect During Live Shadowing Experience
Setting up Email Forwarding
Facilitator Expectations and Grading Help
Changes in Grading Scheme: Incomplete/Complete and 75% to 85%
New (Embedded/Inline Format) How do I grade Course Projects?
Navigating the Gradebook and Accessing the Speedgrader
Quick Reference: Sort assignments in the Speedgrader
How do I Grade Quizzes?
(Old format) How Do I Grade Course Projects and Add Annotations?
Rubrics for Effective Facilitation
Is There an Answer Key for my Course?
How to Monitor and Promote Student Progress
How Do I Grade Discussions?
Adding an Attempt to a Course Project
How Quickly Do I Need to Provide Grading to Students?
Payroll and the Monthly Scheduling Process
Codio References
Manually Graded “Reflect and Submit” Codio Exercises
Codio Quick Resources
Codio Reference: Embedded quiz questions (H5P)
Codio Reference: Checking for Completion Status
Codio Reference: Manually Graded Exercises
Codio Remote Feedback Tools
Codio Reference: Ungraded exercises
Codio Reference: Autograded Exercises
Archived
Table of Contents
- All Categories
- Platform Training
- Course Development & Delivery Platforms
- Codio
- Developers
- Codio Functionality
- Jupyter Notebooks
- Jupyter Notebooks Style Guide
Jupyter Notebooks Style Guide
Updated
by Brock Schmutzler
This article intends to walk you through a consistent style guide for Jupyter Notebooks that is to be applied to all eCornell courses requiring notebooks. By applying consistent practices in notebook materials earlier in courses, it will create a consistent user experience in eCornell courses and save course developers time downstream. The presentation is divided into two parts, the first explaining the appropriate structure of notebooks themselves and the second describing the Markdown tools to do so. The application of this style guide can be seen in this example.ipynb notebook from one of the existing eCornell courses.
Project_One_Name_of_the_Project.ipynb
(or Name_of_Project.ipynb
) would be a good notebook name in a Codio unit called Project One — Name of the Project (or Name of Project) that is embedded on a Canvas page titled Course Project, Part One — Longer Name of the Project.Part 1. Notebook Narrative Structure
Jupyter Notebooks are more than just a means to an end to make scripts more legible. They are a storytelling device for data and workflows and should be treated as such. With that in mind, eCornell course developers working with Jupyter Notebooks (hereafter simply "notebooks") should encourage faculty to adhere to this style guide.
Cell Types
Jupyter uses two kinds of cells, Code and Markdown. Code cells contain code that can be executed with the specified language kernel, and Markdown cells use markdown-formatted text to convey some kinds of ideas. Much like this HelpDocs article, the plain-text is the equivalent of a Markdown cell in Jupyter, which can have various kinds of text formatting, such as bold, italics, fixed-width
, lists, and tables. Conversely, code blocks are the equivalent of notebook Code cells, with the additional feature that Code cells are executable within the notebook and may produce output.
Bearing that in mind, there shouldn't be large comment-blocks in code cells because most (if not all) of the commentary and narrative should be written in Markdown cells above the Code cells. Let's look at examples to illustrate the point.
Bad Example 1: Instructions or Exposition as Code Comments
# first we create a list of numbers, 0-49
# then we take the cumulative sum of them and divide by the sum
# this will give you the (some fake coefficient) of the numerical vector
# keep in mind there are more efficient ways of doing this calculation
import numpy as np
a = range(50)
cs = np.cumsum(a)
ceoff = cs / sum(a)
This Code cell does not conform to the appropriate eCornell style, which stipulates that instructions and exposition should be provided before a Code cell. Instructions and annotations should be treated as equally meaningful as the code it intends to provide context to. Instead, this Code cell should be rewritten as a Markdown cell followed by a Code cell. For example, the Markdown syntax below
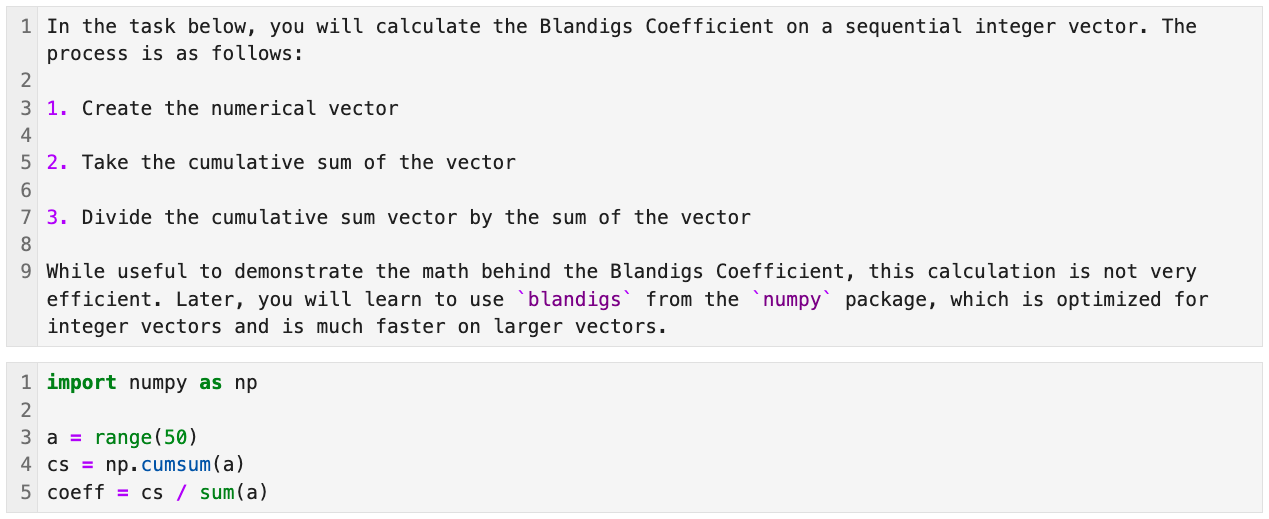
is rendered like this:
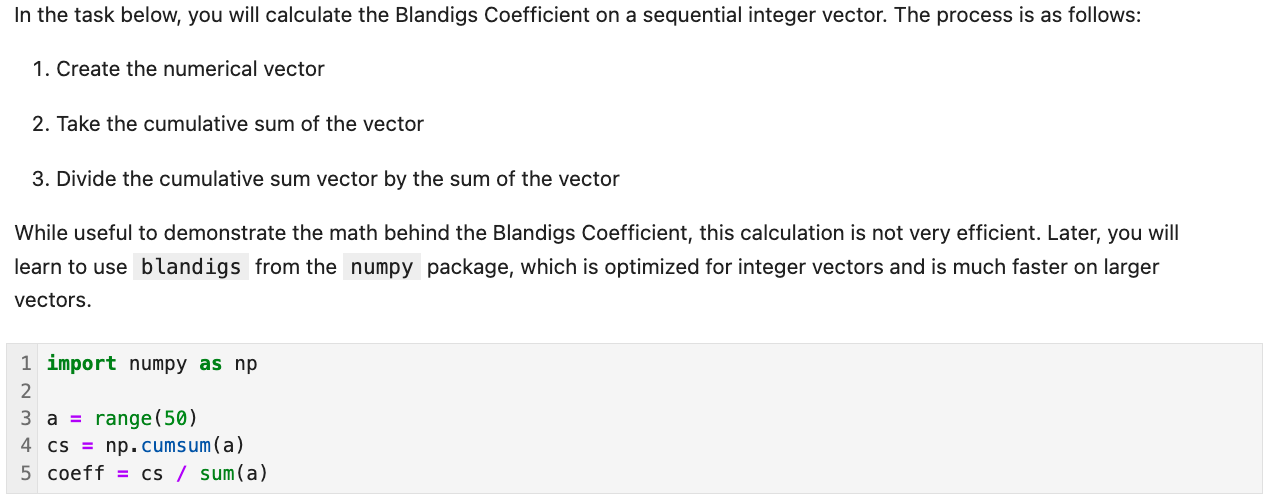
As you can see in the sample above, the Code cell features code and the narrative is restricted to the Markdown cell above. This reduces complex ideas to digestible pieces. In addition, Code cells become more amenable to copy-pasting elsewhere.
Bad Example 2: Intermittent Comments
Another (albeit less egregious) example of a practice to avoid is intermittent comments explaining each step (below). Line numbers in notebooks could be activated in Jupyter, so even this information could be written above in a Markdown cell with a reference to the line number. If this is the first exposure a student has to a particular workflow, then the individual steps should merit their own cells, however if this is later in a course module and the students have already become accustomed to earlier parts of a workflow, inline annotations like the cell below are permissible for brevity.
# import the numpy package
import numpy as np
# generate the sequential integer vector
a = range(50)
# calculate the cumulative sum
cs = np.cumsum(a)
# divide by sum to get the coefficient
ceoff = cs / sum(a)
We can instead rewrite this again as separate markdown and code cells:
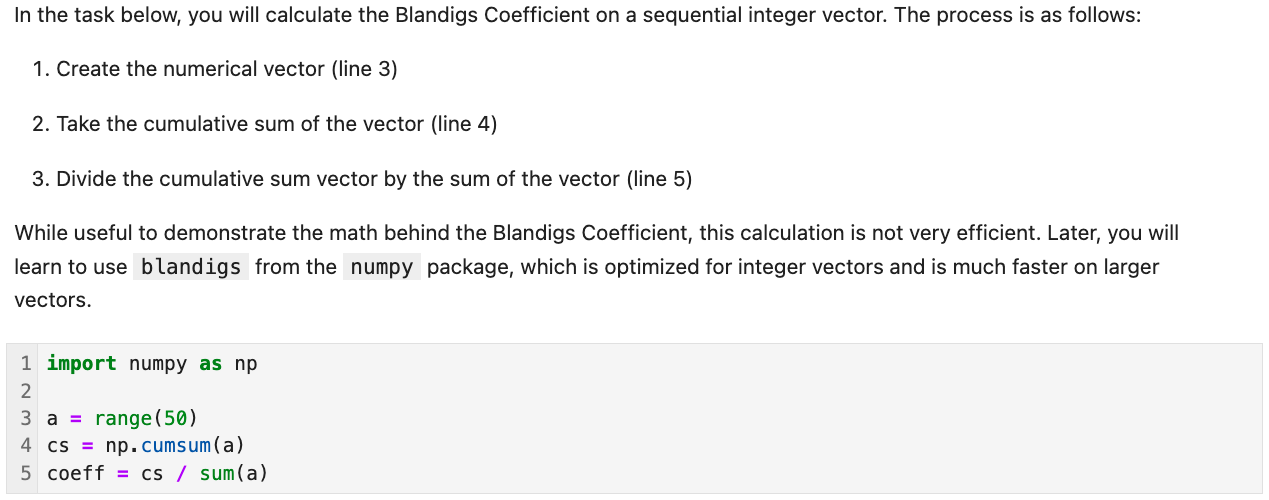
Other Considerations
Types of Code Comments
If using in-code comments as annotations, put code comments above the line of code they are annotating, not inline after it:
avoid
a = range(50) # generate the sequential integer vector
cs = np.cumsum(a) # calculate the cumulative sum
ceoff = cs / sum(a) # divide by sum to get the coefficient
do this instead
# generate the sequential integer vector
a = range(50)
# calculate the cumulative sum
cs = np.cumsum(a)
# divide by sum to get the coefficient
ceoff = cs / sum(a)
Avoid Consecutive Code Cells
When a notebook has consecutive Code cells, there is often times little to no narrative context for Code cells after the first one. Unless a Code cell's purpose is totally clear from previous work in a notebook, all Code cells in the notebook should be accompanied by narrative context.
Notebooks Are Standalone
Each notebook is a standalone assignment/practice/demo/etc. A course using notebooks should not reuse large monolithic notebooks. Students should not be instructed to scroll down to "Section X" and run those cells, or rerun all cells before that starting point. Notebooks start from the top and finish at the bottom, there should not be any parachuting. If assignments or practices rely on the results of previous assignments that have time-consuming processing steps, then save and import the working environment across assignments.
Saving Environments
R allows users to save their workspace as .Rdata
files, which is a binary serialization of all the variables in the active workspace. These .Rdata
files can be sources at the top of a notebook/assignment to import objects from previous work.
Python can serialize objects using the pickle package, which can act similar to .Rdata
serialization. However, you may instead want to save the kernel state and import it between assignments. To accomplish this, use the dill package.
Embrace Headers
Use headers liberally since notebooks have integrated table of contents that allow students jump between sections of notebooks. See below for header formatting in Markdown.
Prioritize Markdown Over HTML
Notebooks have excellent integration with the Markdown language. Use Markdown syntax in all cases where it applies, and supplement Markdown with HTML if you need extra formatting it doesn't provide.
Load Packages Separately
In Julia, Python, and R (for example), best-practice for idiomatic writing is to import packages at the top of a script or notebook, separate from other code. In notebook format, these import statements should live in their own code cell towards the top of the document, after the preamble/introduction (when possible or reasonable to do so). Packages should not be imported mid-document. If necessary, annotate the import calls and explain to students what functionality that package will be providing. This annotation may be useful the first few times the package is called and they are becoming familiar with it, but not necessary afterwards.
Enforce Fixed-Width Formatting
When referencing functions, methods, files, URLs, or specific strings, use fixed-width
formatting (backticks in Markdown). Any item with programmatic relevance must be fixed-width formatted.
Part 2. Leveraging Markdown
Markdown is a very trimmed down syntax that uses just a handful of formatting rules to mark up text for nice HTML rendering. Markdown is preferred in notebooks over HTML, so only use HTML if you require a formatting that isn't possible in Markdown. The basics of the official Markdown cheat sheet are covered in the subsections below.
Headers
Just like HTML <h1>
through <h4>
classes, Markdown uses pound signs #
through ####
to assign header levels, where the number of consecutive pound signs indicates the header level. For example, the HTML syntax <h2>Header Level 2</h2>
is equivalent to ## Header Level Two
in Markdown syntax. The title of your notebook should be a level one header (i.e., # Title
) and all other headers should be level two or lower. DO NOT SKIP HEADER LEVELS! Header levels should always be nested so that level three headers are directly below level two headers and level four headers are directly below level three headers (headers below level four are not recommended and should not be necessary). Nesting headers in this manner will ensure proper header structure, which is particularly important for people using assistive technology because it gives the notebook an intelligible organization.
Lists
Lists can be ordered or unordered.
Unordered
Use hyphens -
to create unordered lists. For example, the syntax
- Item 1
- Item 2
- Item 3
is rendered as
- Item 1
- Item 2
- Item 3
Ordered
Ordered (numbered) lists are automatically numbered. For example, the syntax
1. Item 1
2. Item 2
3. Item 3
will render as:
- Item 1
- Item 2
- Item 3
A great feature is that you can mess up numbering and the Markdown will still render numbers sequentially. For example, the syntax
1. Item 1
3. Item 2
2. Item 3
will still render as:
- Item 1
- Item 2
- Item 3
Formatting
Markdown gives you access to italics (flanking asterisk *italics*
or underscore _italics_
), bold (flanking double-asterisk **bold**
or double-underscore __bold__
), and fixed-width (flanking backticks `fixed-width`
) formatting. For example,
**This is** a *sentence* trying to prove a point about the function `range(1, 50)`.
is rendered as:

Code blocks
To create code blocks, use triple backticks. You can also specify the language the Markdown renderer should use for syntax highlighting.
```python
a = range(1,50)
# now print the scaled results
[print(i + 3.3) for i in a]
```
is rendered as:
a = range(1,50)
# now print the scaled results
[print(i + 3.3) for i in a]
Tables
There's a dirt-simple syntax for nice tables in markdown. You just separate columns with pipes |
. The first row is column titles, the second is the alignment type (left, center, right) depending on where the colon is/are relative to the dashes. Best part is, the text doesn't need to look nicely aligned or formatted.
| left-aligned column | center column | right-aligned column |
| :--- | :---: | ---: |
| some kind of text | potatoes and carrots | a longer desciption here that can drag on for a bit |
| new text | cranberries | the quick brown fox jumps over the lazy dog |
which renders as:

Math
Use flanking dollar signs ($
) to create LaTeX math formatting. For example,
You will need to adjust $x$ by controlling for sample size $n$ using the equation $x\times(1-\frac{n}{15})$.
will render as:

You can use double-dollar signs ($$
) to create a LaTeX math block. For example,
When $a \ne 0$, there are two solutions to a quadratic equation of the form $ax^2 + bx + c = 0$, given by the **quadratic formula**:
$$
x = \frac{-b \pm \sqrt{b^2 - 4ac}}{2a}
$$
will render as:
