Departmental Standards
Company-Wide
eCornell Styleguide & Branding
Cornell University Branding
Writing and Editing Style Guide
Faculty and Expert Naming Conventions in Courses
Cornell School and Unit Names
Tips for Campus Engagements
LSG
Legal Policies
CSG
Updating Wrike Due Dates
Photography Style Guide
eCornell Mini Visual Style Guide
The Pocket Guide to Multimedia Design Thinking (*as It Pertains to Your Job Here)
Creative Services (CSG) Handbook
Administrative
LSG Meeting Recordings and Notes
Sending Faculty Sign-Off Forms in Adobe Sign
Weekly Faculty Status Emails
Animation/Motion Design
Instructional Design
Required Course Elements
The Pocket Guide to Instructional Design Thinking at eCornell
Adding AER to Canvas
Grading
D&D Newsletter
LSG Newsletter (LSGN) - February 2024
LSG Newsletter (LSGN) - March 2022 Edition
LSG Newsletter (LSGN) - December 2023
LSG Newsletter (LSGN) - October 2021 Edition
LSG Newsletter (LSGN) - June 2022 Edition
D&D Newsletter November 2024
LSG Newsletter (LSGN) - August 2022 Edition
LSG Newsletter (LSGN) - June 2023
LSGN Newsletter April 2023
LSG Newsletter (LSGN) - February 2022 Edition
LSG Newsletter (LSGN) - October 2022 Edition
LSGN Newsletter February 2023
LSGN Newsletter March 2023
D&D Newsletter September 2024
LSG Newsletter (LSGN) - August 2023
LSG Newsletter (LSGN) - March 2024
LSG Newsletter (LSGN) - April 2022 Edition
D&D Newsletter - August 2024
LSGN Newsletter January 2023
LSG Newsletter (LSGN) - October 2023 article
LSGN Newsletter (LSGN) - April 2024
LSG Newsletter (LSGN) - November 2021 Edition
D&D Newsletter February 2025
LSG Newsletter (LSGN) - January 2022 Edition
LSGN Newsletter December 2022
D&D Newsletter April 2025
LSG Newsletter (LSGN) - July 2022 Edition
LSG Newsletter (LSGN) - September 2022 Edition
Course Development
Image Uploads for Inline Projects
How to Install the Firefox Canvas Utilities Extension
Revising a Course/ Creating a Redux Version/ Course Updates
Creating a Perma Link With Perma.cc
Course Content Deletion Utility — Removing All Course Content
Teleprompter Slide Template
Course Names
Requesting High Resolution Video Uploads
Technical Talking Points Template
Online Resources in Credit-Bearing Courses
Hiring Actors for an eCornell Project
Marketing
Operations
Tech
Master Course Template Differences (8675309s)
Doc-Based Master Course Template and Standards (8675309-DOC)
Pedagogical Guidelines for Implementing AI-Based Interactives: AER
Coding Master Course Template and Standards (8675309-CODE)
Hero Image
Platform Training
Administrative Systems
ADP
Google Drive
Downloadables Process
Embed a Document from Google Drive
Adding Google Links to Canvas
File Naming and Storage Convention Standards
Google Drive for Desktop Instructions
Storing Documents in Multiple Locations
Wrike
Wrike System Fundamentals
Field Population
1.0 to 2.0 Wrike Project Conversion
Blocking Time Off in Work Schedule (Wrike)
Wrike Custom Field Glossary
Wrike "Custom Item Type" Definitions
How to Create a Private Dashboard in Wrike
Using Timesheets in Wrike
Importing Tasks into a Wrike Project
Wrike Project Delay Causes Definitions
Setting OOO Coverage for Roles in Wrike
How to Change a Project's Item Type in Wrike
Using Search in Wrike
How to Create a Custom Report in Wrike
@ Mentioning Roles in Wrike
Automate Rules
Using Filters in Wrike
Managing Exec Ed Programs in Wrike
External Collaborators
Wrike for External Collaborators: Getting Started
Wrike for External Collaborators: Views
Wrike for External Collaborators: Tasks in Detail
Wrike Updates
New Experience Update in Wrike
Wrike Course Development Template 2.0 - What's New
Wrike - Course Development Template 3.0 Release Notes
Wrike Process Training
Course Development & Delivery Platforms
Canvas
Development
Adding Custom Links to Course Navigation
Adding Comments to PDFs from Canvas Page Links
Setting Module Prerequisites and Requirements in Canvas
Canvas Page Functionality
Create a New Course Shell From 8675309
Using LaTeX in Canvas
Search in Canvas Using API Utilities - Tutorial
Reverting a Page to a Previous Version
Student Groups
Create Different Canvas Pages
Importing Specific Parts of a Canvas Course
Canvas HTML Allowlist/Whitelist
Understanding Canvas Customizations/Stylesheets
Operations
Discussion Page Standards
How to import a CU course containing NEW quizzes
Canvas LMS: NEW Quiz compatibility
Faculty Journal
Course Content Style Guide
Click-To-Reveal Accordions in Canvas
Course Maintenance Issue Resolution Process
Meet the Experts
Codio
Codio Operations
Managing Manually Graded “Reflect and Submit” Codio Exercises
Codio Structure and Grading for Facilitators
Premade Codio Docs for Ops & Facilitators
Codio Remote Feedback Tools for Facilitators
Developers
Development Processes
Creating a New Codio Course
Creating a New Codio Unit
Integrating a Codio Course into Canvas
Embedding a Codio Unit into Canvas
Setting Up the Class Fork
Setting Up the Class Fork (LTI 1.3)
R Studio - Exclusion List for R Code
Mocha/Selenium Autograding
Starter Packs in Codio
Configuring Partial Point Autograders in Codio
Launch a Jupyter Notebook from VM
Program-Specific Developer Notes
Codio Functionality
Jupyter Notebooks
Jupyter Notebooks - nbgrader tweaks
Jupyter Notebooks Style Guide
Adding Extensions to Jupyter Notebooks
Setting up R with Jupyter Notebooks
Change Jupyter Notebook Auto Save Interval
How to Change CSS in Jupyter Notebook
RStudio in Codio
How To Centralize the .codio-menu File to One Location
Codio Fundamentals for LSG
Using the JupyterLab Starter Pack
Using Code Formatters
Using the RStudio Starter Pack
Conda Environments in Codio
Updating Codio Change Log
eC Facilitator Guide to Codio
Migrating to Updated Codio Courses
Qualtrics
Ally
Ally Institutional Report Training
Ally Features Overview Training
Using the Ally Report in a Course
Ally Vendor Documentation/Training Links
Adobe
Other Integrations
H5P
Modifying Subtitles in H5P Interactive Videos
Embedding H5P Content Into Canvas
Troubleshooting H5P Elements in Canvas
Inserting Kaltura Videos into H5P Interactive Videos
Adding Subtitles to H5P Interactive Videos
S3
BugHerd
Instructional Technologies & Tools Inventory
Canvas API Utilities
Getting started with the MOP Bot
eCornell Platform Architecture
HR & Training Systems
Product Development Processes
Accessibility
What Is Accessibility?
What Is Accessibility?
Accessibility Resources
Accessibility Considerations
Accessibility Support and Assistive Technology
Structural Accessibility
Accessibility Design and Development Best Practices
Accessible Images Using Alt Text and Long Descriptions
Accessible Excel Files
Accessibility and Semantic Headings
Accessible Hyperlinks
Accessible Tables
Creating Accessible Microsoft Files
Mathpix: Accessible STEM
Design and Development General Approach to Accessibility
Integrating Content Authored by a Third Party
Planning for Accessible Tools
Accessibility Considerations for Third Party Tools
Studio Accessibility
Designing for Accessible Canvas Courses
Accessibility: Ongoing Innovations
Course Development
Planning
Development
0. Design
1. Codio Units
1. Non-Video Assets
3. Glossary
4. Canvas Text
4. Tools
4. Tools - Wrike Task Definitions
3. Review And Revise Styled Assets
ID/A to Creative Team Handoff Steps
General Overview of Downloadables Process
Course Project: Draft and Final
Excel Tools: Draft and Final
eCornell LSG HTML Basics
1. Non-Video Assets - Wrike Task Definitions
2. Video
Multifeed Video
2. Video (Standard) - Wrike Task Definitions
Studio Tips
Tips for Remote Video Recording Sessions
Who to Tag for Video Tasks
3. Animation
3. Animation - Wrike Task Definitions
2. Artboard Collab Doc Prep
6b. Motion Design Review and Revise
Who to Tag for Animations Tasks
3. Artboard Collab Process Walkthrough
DRAFT - FrameIO Process Walkthrough
Motion Contractor Guide for IDAs / IDDs
Requesting / Using Stock Imagery (Getty Images and Shutterstock)
3. Ask the Experts
5. On-Demand Conversion
1. Write Content for On-Demand
On-Demand: Conversion Notes
On-Demand: Writing Quiz Questions
On-Demand: Writing Blended Learning Guides (DRAFT)
On-Demand: Lesson Description and Objectives (DRAFT)
2. Build On-Demand Lesson
On-Demand: Create a Blended Learning Guide (BLG)
On-Demand: Create Lesson Shells in Canvas
On-Demand: Populate Homepage Content
On-Demand: Add Quiz Assessment Content
On-Demand: Reformat Wrap-Up
On-Demand: Prepare Lesson for QA
On-Demand: Request Banner Image
OD Updates Process
5. On-Demand Conversion - Wrike Task Definitions
5. Review
5. Review - Wrike Task Definitions
1. Prep Course for Reviews
2. Conduct Student Experience Review
3. Implement Creative Director Edits
3. Implement IDD Edits
3. Implement Student Experience Review Edits
4. CSG - Revise Tools Export 1
5. Conduct Faculty Review
6. Implement Faculty Edits
7. Conduct Technical Review of Course (STEM-only)
2. Conduct IDD or Sr ID Review
6. Alpha
6. Alpha - Wrike Task Definitions
Alpha Review Process
Prepare a course for Alpha review
Schedule & Conduct Alpha Triage Meeting
7. QA
7. QA - Wrike Task Definitions
1. Complete Dev to QA Checklist
2. Copy Edit Captions
2. Copy Edit Course & Files
4. Conduct Content QA of Course
4. Final Creative Review and Export
Adding Chat With Tech Support to Course Navigation
5. Implement QA Edits
Working With Video Captions That Contain Special Characters
Copy Edit Captions in SubPLY
Creating a Course Style and Settings Guide
Copy Editing Content in Frame.io
Copy Edit Captions in 3Play
Tag a Video for Transcription by 3Play
Course QA Checklists
8. Deployment
8. Deployment - Wrike Task Definitions
1. Finalize Master Version of Course
2. Create & Add Course Transcript (CT) to Course
Replace a Master -M With a Redux Version of the Course
3. Create -T (Training Course) and Associate With Master Blueprint
Canvas Blueprint Course Functionality
Project Management in Wrike
Managing Project Reporting in Wrike
Managing Task Needs/Schedule in Wrike
Adding Tasks
Comments and Communication
Statuses
Updating Task Start and Due Dates
Predecessors
Durations
Rollups
Calculating Project Schedule by Deadline in Wrike
Creating Course Project Plans in Wrike
Setting Custom Capacity for Resources
Customizing Effort in a New Project Plan
Marking Projects Complete in Wrike
How to Set Up Workload Charts to Track Effort in Wrike
For-Credit Considerations
1-Sheet Population
Post-Development
Program Facilitation & Operational Guidelines
Data Science
Facilitator Resources
Canvas Navigation
Adding Events to the Course Calendar
Navigating Canvas and the Dashboard
How Do I View Previous Courses I Have Taken or Facilitated?
Why Am I Receiving Duplicate Canvas Emails?
How Do I Edit My Canvas Profile?
Send Students a Direct Message on Canvas
Adding Notes to Canvas Gradebook
How Do I Send Students Nudges from the Gradebook?
Overview of 2024 Changes- Course Layout and Structure
How Can I Update My Canvas Notification Preferences?
Ursus Navigation
How Do I Access My Offer in Ursus?
How Do I Edit My Ursus Profile?
How Do I Request Time Off (Blackout Dates)?
How Can I Request to Learn More Courses?
Live Sessions
When Should I Schedule My Live Sessions For?
Changing Live Session Date and Time After Created with Facil Tool
Help! I Need to Reschedule a Live Session
Live Session Information page
Set up Live Sessions with the Facil Tool
Combining Live Sessions with Facil Tool
Course Set-Up
Course Set Up: Getting Ready for Live Sessions
Recording and Posting a Welcome Video
Course Set Up: When can I begin to edit my course?
Course Set Up: Reviewing Due Dates
Course Set Up: Reviewing Announcements
What Do I Need to Do to Make Sure My Course is Set Up Correctly?
How Do I Customize My Course Sections?
Course Announcements and Messages Templates
Facilitator Focus
Zoom and other Technical Support
How Do I Set Up My Zoom Account?
Support Resources for Facilitators
Zoom Features: Preparing for Live Sessions
How to Upload Videos to Zoom On-Demand
Student Survey FAQs
How Do I Save and Refer Back to Zoom Recordings?
How Do I Find My Personal ID Meeting link in Zoom?
Benefits as an eCornell Employee
Do I Have Access to Microsoft Office as a Cornell Employee?
Taking Courses as a Student
Professional Development Benefit
Student Success
Help! My Students Can't View a Video Within My Course
Extensions and Retakes
Policies and Navigation Resources for Students
Students enrolled through special groups: Corporate and VA
Unique Circumstances for Student Extensions and Retakes
Can I Provide Students with a Letter of Recommendation?
Understanding and Addressing Instances of Plagiarism
Support Resources for Students
Understanding and Addressing Use of AI
Help! My Student is Having a Hard Time Uploading a Video
Unique Student Situations
I Have a Student Requesting Accommodations- How Should I Proceed?
Canvas Mobile App
Messaging Students via the Canvas Mobile App
Setting up and Navigating the Canvas Mobile app
Setting up Push Notifications on Canvas Mobile
Canvas App Features for eCornell courses
New Facilitator Onboarding and Quick References
Facilitator Onboarding at eCornell
Why Do I Have Multiple eCornell email and Canvas Accounts?
Quick reference: Systems and Accounts we use at eCornell
Quick reference guide: Key eCornell Personnel
How do I log Onto Canvas and Access FACT101?
How Do I Add eCornell to My Email Signature?
Getting the Most Out of Learning Assignments
What to Expect During Live Shadowing Experience
Setting up Email Forwarding
Facilitator Expectations and Grading Help
Changes in Grading Scheme: Incomplete/Complete and 75% to 85%
New (Embedded/Inline Format) How do I grade Course Projects?
Navigating the Gradebook and Accessing the Speedgrader
Quick Reference: Sort assignments in the Speedgrader
How do I Grade Quizzes?
(Old format) How Do I Grade Course Projects and Add Annotations?
Rubrics for Effective Facilitation
Is There an Answer Key for my Course?
How to Monitor and Promote Student Progress
How Do I Grade Discussions?
Adding an Attempt to a Course Project
How Quickly Do I Need to Provide Grading to Students?
Payroll and the Monthly Scheduling Process
Codio References
Manually Graded “Reflect and Submit” Codio Exercises
Codio Quick Resources
Codio Reference: Embedded quiz questions (H5P)
Codio Reference: Checking for Completion Status
Codio Reference: Manually Graded Exercises
Codio Remote Feedback Tools
Codio Reference: Ungraded exercises
Codio Reference: Autograded Exercises
Archived
- All Categories
- Platform Training
- Course Development & Delivery Platforms
- Codio
- Developers
- Development Processes
- Mocha/Selenium Autograding
Mocha/Selenium Autograding
Updated
by Nick
What is Mocha?
Mocha is a feature-rich JavaScript test framework running on Node.js and in the browser, making asynchronous testing simple to run. Mocha tests run serially, allowing for flexible and accurate reporting, while mapping uncaught exceptions to the correct test cases.
What is Selenium?
Selenium is an open-source suite of tools and libraries that is used for browser automation. Selenium is used to:
- Allow users to test their websites functionally on different browsers.
- Perform Cross browser testing to check if the website functions consistently across different browsers.
It provides a single interface that lets you write test scripts in programming languages like Ruby, Java, NodeJS, PHP, Perl, Python, JavaScript, and C#, among others. Selenium is very extensible and can be integrated with other tools and frameworks like TestNG, JUnit, Cucumber, etc.
For web-based projects, we can use the Selenium Webdriver to drive a browser natively, as a user would, either locally or on a remote machine using the Selenium server.
JavaScript Development
Starter Pack: Mocha/Selenium JS Auto Grading
Stack: Mocha/Selenium Auto Grading
In Codio, we'll want to use Headless Chrome for developing auto graded tests. Headless Chrome lets you run the browser in an unattended environment without any visible UI.
To start off our auto graded tests, we'll need a few lines of code in our script to require Headless Chrome, the Selenium WebDriver and the assert library that will be used in our tests. The assert libraries provide developers with a set of tools to validate the behavior and output of their code. They enable the creation of expressive and human-readable test cases that can affirm whether a given piece of code behaves as intended.
We'll begin with the following lines of code:
const {Options} = require('selenium-webdriver/chrome');
const options = new Options();
const {By, Builder} = require('selenium-webdriver');
const assert = require("assert");
Next, we need to set up the test suite, which can support multiple tests along with a before function. The before function is an asynchronous function which will simulate the activity the user/student will be doing when interacting with the webpage. Important Note: Using this method, as long as the solution code is correct, any and all interactivity done for the purpose of autograding will take place using the before function's code. Neither the student nor the facilitator will need to manually interact with the web page in order to successfully run an auto graded test.
The following code is the outer layer code which while house the individual tests:
describe('Assessment returned that - ', function () {
this.timeout(0); // No time limit
let driver;
// Setting up the driver and declaring the test file to be used in the test suite
// Simulate student interactivity - user actions OR to make an item accessible
before(async function () {
driver = await new Builder().forBrowser('chrome').setChromeOptions(
// Uncommment the next line before publishing, otherwise it will stop the browser. Commenting out is ONLY used for debugging
options.addArguments('--headless=new')
).build();
await driver.get('file:///home/codio/workspace/exercise_files/index.html');
// opens the item we want to focus on ...so then we can get info from it (ID per element, etc)
//Find the element by it's ID called "openGallery"
let openGalleryBtn = await driver.findElement(By.id('openGallery'));
//Simulate a click on the button we just found
await openGalleryBtn.click();
//We can add additional interactive steps here as needed
});
// Clean the execution
// Uncommment the next line before publishing, otherwise it will stop the browser. Commenting out is ONLY used for debugging
after(async () => await driver.quit()); // Closes the browser
});
Important note:
Note the following lines that are in the code block above:
options.addArguments('--headless=new')
and
after(async () => await driver.quit()); // Closes the browser
These two lines must be uncommented before publishing the Codio unit. These two lines are only to be commented out during the debug process. Debugging will be discussed later in this article.
Now, considering the next line:
await driver.get('file:///home/codio/workspace/exercise_files/index.html');
Simply change the file path in this line to the file that needs to be tested.
Now we are ready to add our individual unit tests to our auto graded script. We will be using the revised CIS560s course for our example tests. In the examples used, we will be using an application that when clicking a button, opens up a modal lightbox for an image gallery. Please see the example below:
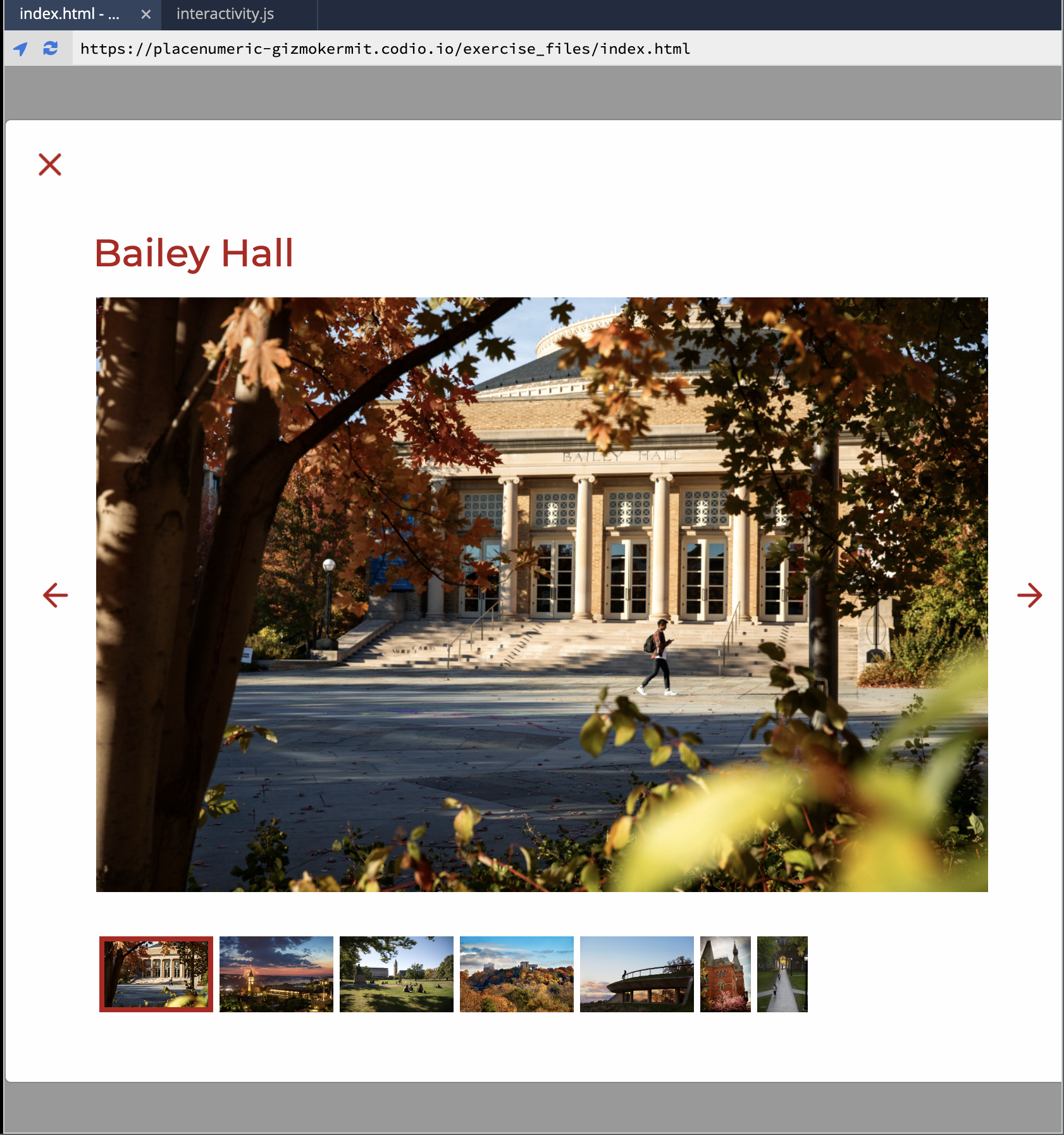
Let's begin our first unit test:
it('The "thumbnail" class is selected', async function () {
// Search for the ribbon
let ribbon = await driver.findElement(By.id("thumbnailRibbon"));
// Get all elements with the tag "img" inside the ribbon
let images = await ribbon.findElements(By.tagName("img")).then(async function(elements){
// Simulate a click on any of the elements
elements[2].click();
// Get the class attribute of the element
let elementClasses = await elements[2].getAttribute("class");
// Now, assert the class of the element that was clicked is "selected"
assert.match(elementClasses, /selected/); // Match selected
});
})
As seen from the snippet above, we can do specific simulated actions pertaining to each individual test. Once we get the image ribbon stored in our images object, we can simulate a click on any of the elements just like a student user would do. At the very end, we use the assert library by doing an assert.match() to test if the class of the element is "selected". Node.js handles the assert statements. We can find the full list of possible assert statements here.
At the very top of the snippet, we have the it() function, followed by a string as the first parameter. Whatever is written in the string, will be what shows to the user to denote what the test is trying to assert in readable text.
The following is an example of what will be displayed to the student after clicking the "Test Code" button:
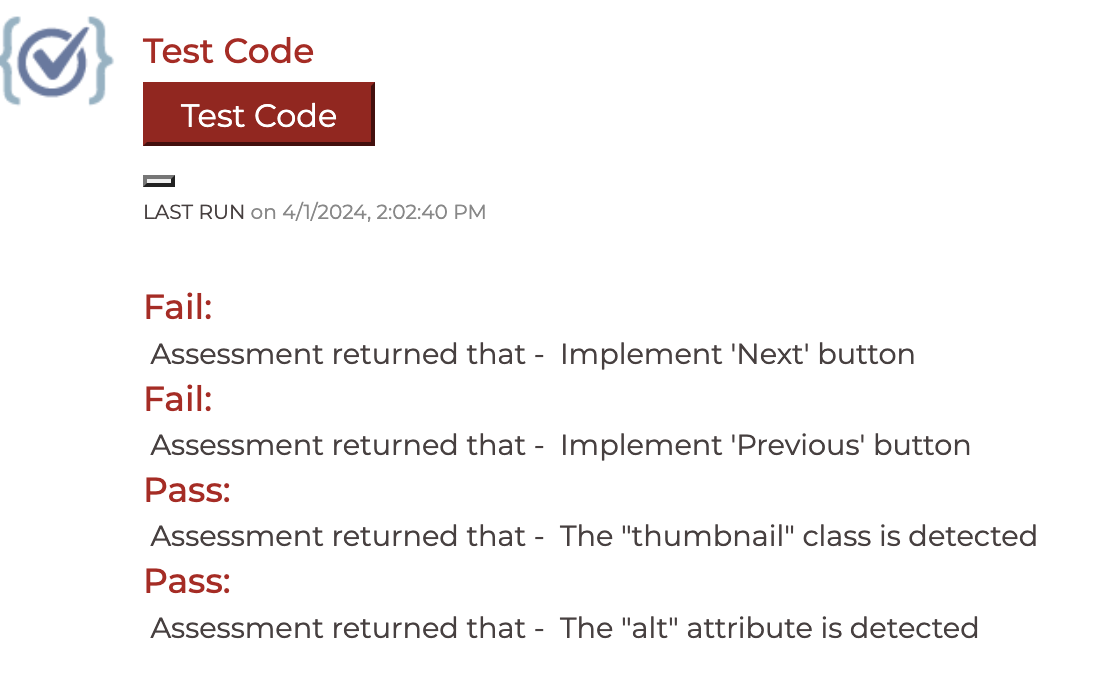
As we can see from the image above, the first two tests have failed, while the second two tests have passed. The student will receive 50% as their score from this assessment.
Setting up the Codio JavaScript Unit
- Configure the preview button in the Codio menu. After the preview button is configured, we can click the button newly to be named "Mocha" to run the auto grader first inside the Codio terminal for development purposes. To enable this, make sure the following code is present:
{
// Configure your Run and Preview buttons here.
// Run button configuration
"commands": {
"Mocha": "mocha .guides/tests/testJS.js --reporter .guides/secure/score.js 2>&1 || true"
},
// Preview button configuration
"preview": {
"Project Index (static)": "https://{{domain}}/{{index}}",
"Current File (static)": "https://{{domain}}/{{filepath}}",
"Box URL": "https://{{domain3000}}/"
}
}
To run the unit tests inside the terminal without the reporter, the following command only should be run:
mocha .guides/tests/testJS.js
- Set up the reporter. Under .guides/secure/, add a file called "score.js", for example. and paste in the following script. The reporter does two things. It passes the grade the student receives from the automated tests as well as presents the output in a clean format that we're able to manage in the file.
- Set up the package.json file. Create this file in the /workspace directory with the following code pasted in:
{
"scripts": {
"test": "mocha .guides/tests/testJS.js --reporter .guides/score.js 2>&1 || true"
},
"dependencies": {
"assert": "^2.0.0",
"chai": "^4.3.10",
"chai-dom": "^1.11.0",
"jsdom": "^22.1.0",
"jsdom-global": "^3.0.2",
"selenium-webdriver": "^4.14.0"
},
"devDependencies": {
"mocha": "^10.2.0"
}
}
Using the terminal command npm install, the above dependancies will be installed.
- Create the Advanced Code Test. The command to run will be "npm test" and partial points will need to be turned on if there are more than one test to be run.
Debugging JavaScript Code with Mocha and Selenium
- First, in the auto grade script, comment out the following two lines:
options.addArguments('--headless=new')
after(async () => await driver.quit()); // Closes the browser
- In the Codio menu, click on Project Index (Static) and change it to Box Url. A new tab will appear showing a subdomain name along with a port number (3000).
- Change the port number in the URL from 3000 to 3050. Doing this will allow you to interact with the browser that Selenium is using.
- In Codio, click on the Mocha button to open the browser in the new tab. Note: Each time the Mocha button is clicked, it will open a new instance of the Chrome browser. Close each instance of the Chrome browser before opening up a new one with the Mocha button. If there are too many instance of Chrome running in this method, Mocha will run out of memory and fail.
- Now we will be able to interact with the browser just like the code put into the before() function. Note: Selenium will interpret this fairly quickly, so it's possible the use of timers might be needed.